Circular Link List complete code and application
-circular linked list c++ -circular linked list c++ code – circular linked list in data structure -Data structure and algorithm –Data structure and algorithm in C++ – Data structure using C++ -Data structure projects in C++ – Data structure Project idea -Data structure MCQ -Data structure Interview Question –data structure question paper –free course online –past papers -final year projects for computer science with source code -semester project ideas -computer programming -computer science interview questions- tutorial -cui
Basic Operations
Following are the important operations supported by a circular list.
- insert
- delete
- display
For the working detail diagram of these operation click here
Single Circular Link list
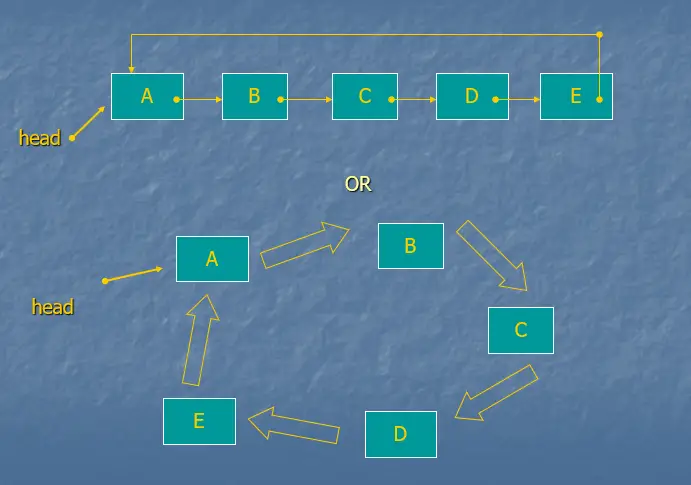
Doubly Ring
Doubly Ring data structure can be regarded as Circular Doubly Link List. In Circular Doubly Link List, instead of making last node’s next pointer to NULL, We assign or point it to head node. Similarly, first (head) node’s previous pointer is pointed to last node in list . so that it sounds as Doubly Ring data structure.
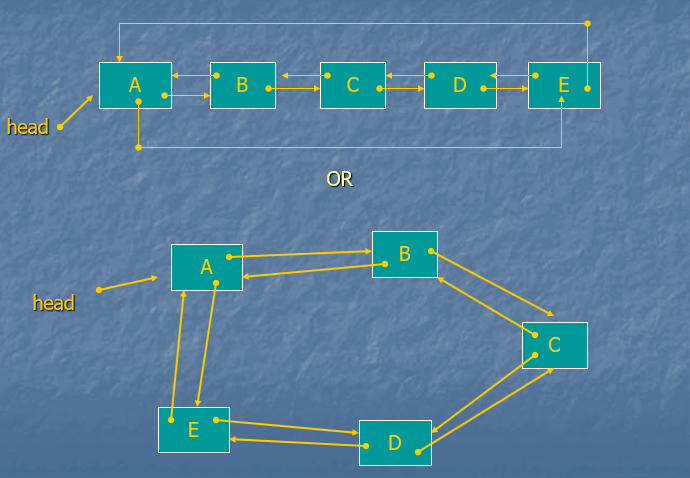
Application of Circular Linked List
- Personal Computers, where multiple applications are running. All the running applications are kept in a circular linked list and the OS gives a fixed time slot to all for running. The Operating System keeps on iterating over the linked list until all the applications are completed.
- Another example can be Multiplayer games. All the Players are kept in a Circular Linked List and the pointer keeps on moving forward as a player’s chance ends.
- Circular Linked List can also be used to create Circular Queue. In a Queue we have to keep two pointers, FRONT and REAR in memory all the time, where as in Circular Linked List, only one pointer is required.
C++ code for Single circular link list
#include<iostream>
#include<cstdio>
#include<cstdlib>
using namespace std;
struct node
{
int info;
struct node *next;
}*p;
class circular_llist
{
public:
void create_node(int value);
void add_after(int value, int position);
void delete_element(int value);
void display_list();
circular_llist()
{
p = NULL;
}
};
int main()
{
int choice, element, position;
circular_llist cl;
while (1)
{
cout << “1.Create Node” << endl;
cout << “2.Add after” << endl;
cout << “3.Delete” << endl;
cout << “4.Display” << endl;
cout << “5.suit” << endl;
cout << “Enter your choice : “;
cin >> choice;
switch (choice)
{
case 1:
cout << “Enter the element: “;
cin >> element;
cl.create_node(element);
cout << endl;
break;
case 2:
cout << “Enter the element: “;
cin >> element;
cout << “Insert element after position: “;
cin >> position;
cl.add_after(element, position);
cout << endl;
break;
case 3:
if (p == NULL)
{
cout << “List is empty, nothing to delete” << endl;
break;
}
cout << “Enter the element for deletion: “;
cin >> element;
cl.delete_element(element);
cout << endl;
break;
case 4:
cl.display_list();
break;
case 5:
exit(1);
break;
default:
cout << “Wrong choice” << endl;
}
}
return 0;
}
//Insert Operation
void circular_llist::create_node(int value)
{
node *t;
t = new node;
t->info = value;
if (p == NULL)
{
p = t;
t->next = p;
}
else
{
t->next = p->next;
p->next = t;
p = t;
}
}
void circular_llist::add_after(int value, int pos)
{
if (p == NULL)
{
cout << “First Create the list.” << endl;
return;
}
node *t, *s;
s = p->next;
for (int i = 0; i < pos – 1; i++)
{
s = s->next;
if (s == p->next)
{
cout << “There are less than “;
cout << pos << ” in the list” << endl;
return;
}
}
t = new node;
t->next = s->next;
t->info = value;
s->next = t;
if (s == p)
{
p = t;
}
}
//delete operation
void circular_llist::delete_element(int value)
{
node *t, *s;
s = p->next;
if (p->next == p && p->info == value)
{
t = p;
p = NULL;
free(t);
return;
}
if (s->info == value)
{
t = s;
p->next = s->next;
free(t);
return;
}
while (s->next != p)
{
if (s->next->info == value)
{
t = s->next;
s->next = t->next;
free(t);
cout << “Element ” << value;
cout << ” deleted from the list” << endl;
return;
}
s = s->next;
}
if (s->next->info == value)
{
t = s->next;
s->next = p->next;
free(t);
p = s;
return;
}
cout << “Element ” << value << ” not found in the list” << endl;
}
//Display List Operation
void circular_llist::display_list()
{
node *s;
if (p == NULL)
{
cout << “List is empty” << endl;
return;
}
s = p->next;
cout << “Circular Link List: = “;
while (s != p)
{
cout << s->info << “->”;
s = s->next;
}
cout << s->info << endl;
cout << endl;
}
Related links
Single link list Stack AVL Trees Binary search Counting Sort
Doubly link list Queue Graphs Bubble Sort Radix sort
Circular link list Binary search tree Hashing Insertion Sort Bucket Sort
Josephus Problem Tree Traversal Heaps Quick Sort Merge Sort
At Cui tutorial, courses, past papers and final year projects
#circular linked list c++ #circular linked list c++ code # circular linked list in data structure #Data structure and algorithm #Data structure and algorithm in C++ # Data structure using C++ #Data structure projects in C++ # Data structure Project idea #Data structure MCQ #Data structure Interview Question #data structure question paper #free course online #past papers #final year projects for computer science with source code #semester project ideas #computer programming #computer science interview questions# tutorial #cui
#courses #pastpaper #Finalyearproject #tutorial #cui #project #programming #computer science