Variables, Expressions and Statements in Python
Variables, Expressions and Statements in Python Programming
The Lecture will introduce students to:
- Variables
- Python Numbers
- Python Strings
- Basic operations on numbers
- Basic operations on strings
Variables in python
Variables are reserved memory locations to store values. This means that when you create a variable you reserve some space in memory. Based on the data type of a variable, the interpreter allocates memory and decides what can be stored in the reserved memory. Therefore, by assigning different data types to variables, you can store integers, decimals or characters in these variables.
Python supports different type of variables such as whole numbers, floating point numbers and text. You do not need to specify the type of variable. You can simply assign any value to a variable.
Literal constants
Literal constants are values that are used as such. Their values do not change.
Example
An example of a literal constant is a number, like 5, 1.23, or a string such as ‘This is a string’ or “It’s a string!” The number 2 always represents itself and nothing else. It is a constant because its value cannot be changed from 2.
Data Types in python
Basic Data Types in Python are:
- Integers : An example of an integer is 2 which is just a whole number.
- Floats : Examples of floating point numbers (or floats) are 3.23, 52.3E-4.
- String : A string is a sequence of characters. They are bunch of words.
Activity 1:
Display numbers on screen using Python IDLE.
Solution:
- Run Python IDLE
- Type in any number, say 24 and press Enter.
- 24 should be printed on the screen
- Now type 2, press Enter.
- 2 should be displayed on screen as an output.
- Now type print(234). Press Enter. 234 will be the
- Type print(45.90) and press Enter. The output will show 45.9 on screen.
Activity 2:
Display strings on screen.
Solution:
- Python recognized strings through quotes (single, double). Anything inside quotes is a string for Python
- Type hello, press Enter. An error message will be displayed as Python interpreter does not understand this as a
- Type ‘hello’ and press Enter. Hello will be displayed.
- Type ‘Quote me on this!’ and press Enter. Same string will be displayed.
- Type “What’s your name?” and press Enter. What’s your name? will be printed on the screen.
- You can specify multi-line strings using triple quotes “ “ “ or ‘ ‘ ‘. Type following text and press Enter.
Multi line string
Activity 3:
Use Python as a calculator.
Solution:
- The interpreter acts as a simple calculator: you can type an expression at it and it will write the Expression syntax is straightforward: the operators + (addition), – (subtraction), * (multiplication) and / (division) work just like in most other languages. Parentheses (()) can be used for grouping.
- Type in 2 + 2 and press Enter. Python will output its result : 4.
- Try following expressions in the shell.
- 50 – 4
- 23.5 – 2.0
- 23 – 18.5
- 5 * 6
- 2.5 * 10
- 2.5 * 2.5
- 28 / 4 (note: division returns a floating point number)
- 26 / 4
- 23.4 / 3.1
Activity 4:
Get an integer answer from division operation. Also get remainder of a division operation in the output.
Solution:
- Division (/) always gives a float. There are different ways to get a whole as an answer.
- Use // operator:
- Type 28 // 4 and press Enter. The answer is a whole
- Type 26 // 4 and press Enter.
- Use int() cast operator: this operator changes the interchangeable
- Type int(26 / 4) and press Enter. The answer is a whole
- Type int(28/4); press Enter.
- The modulus (or mod) % operator is used to get the remainder as an output (division/operator returns the quotient).
- Type 28 % 4. Press Enter. 0 will be the result.
- Type 26 % 4. Press Enter. 2 will be the result.
Activity 5:
Calculate 43, 410, 429, 4150, 41000
Solution:
- The multiplication (*) operator can be used for calculating powers of a number. However, if the power is big, the task will be tedious. For calculating powers of a number, Python uses **.
- Type following and obtain the results of above
- 4 ** 3
- 4 ** 10
- 4 ** 29
- 4 ** 150
- 4 ** 1000
Activity 6:
Write following math expressions. Solve them by hand using operators’ precedence. Calculate their answers using Python. Match the results.
Solution:
The table below shows the operator precedence in Python (from Highest to Lowest).
Operator | Operation | Example |
** | Exponent | 2 ** 3 = 8 |
% | Modulus/remainder | 22 % 8 = 6 |
// | Integer division/floored quotient | 22//8 = 2 |
/ | Division | 22/8 = 2.75 |
* | Multiplication | 3*5 = 15 |
– | Subtraction | 5 – 2 = 3 |
+ | Addition | 2 + 2 = 4 |
Calculate following expressions:
- 2+3*6
- (2+3)*6
- 485 * 57
- (5 – 1) * ((7 + 1) / (3 – 1))
- 42 + 5 * * 2
Activity 7:
Concatenate two or more strings. Also replicate a string.
Solution:
The meaning of an operator may change based on the data types of the values next to it. For example, + is the addition operator when it operates on two integers or floating- point values. However, when + is used on two string values, it joins the strings as the string concatenation operator.
- Enter the following into the interactive shell: ‘Ali’ + ‘Ahmad’. The result will be ‘AliAhmad’
The expression evaluates down to a single, new string value that combines the text of the two strings. However, if you try to use the + operator on a string and an integer value, Python will not know how to handle this, and it will display an error message.
- Type ‘Alice’ + 42 and press Following error will be displayed.
String plus integer
The * operator is used for multiplication when it operates on two integer or floating- point values. But when the * operator is used on one string value and one integer value, it becomes the string replication operator. Enter a string multiplied by a number into the interactive shell to see this in action.
- Type ‘Alice’ * 5 and press The output will be ‘AliceAliceAliceAliceAlice’
The expression evaluates down to a single string value that repeats the original a number of times equal to the integer value. String replication is a useful trick, but it’s not used as often as string concatenation.
The * operator can be used with only two numeric values (for multiplication) or one string value and one integer value (for string replication). Otherwise, Python will just display an error message.
- Type ‘Ali’ * ‘Ahmad’; press enter error will occur.Type ‘Alice’ * 0; press Enter. Following error will occur.
Activity 8:
Use python variables to store values.
Solution:
You’ll store values in variables with an assignment statement.
An assignment statement consists of a variable name, an equal sign (called the assignment operator), and the value to be stored.
If you enter the assignment statement spam = 42, then a variable named spam will have the integer value 42 stored in it.
Enter the following into the interactive shell.
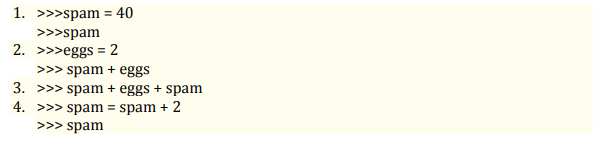
A variable is initialized (or created) the first time a value is stored in it.
After that, you can use it in expressions with other variables and values.
When a variable is assigned a new value ③, the old value is forgotten, which is why spam evaluated to 42 instead of 40 at the end of the example. This is called overwriting the variable. Enter the following code into the interactive shell to try overwriting a string:
>>> spam = ‘Hello’
>>> print(spam)
>>>spam = ‘Goodbye’
>>print(spam)
Python allows you to assign a single value to several variables simultaneously.
>>> a = b = c = 100
>>>a
>>>b
>>>c
>>>print(a,b,c)
>>>a, b, c = 1, 2, “John”
>>>print(a,b,c)
Activity 9:
Try different variable names and check the output.
Solution:
You can name a variable anything as long as it obeys the following three rules:
- It can be only one
- It can use only letters, numbers, and the underscore (_)
- It can’t begin with a number.
Try following statements in Python:
- Balance = 0
- Current-balance = 4
- currentBalance = 1
- current_balance = 28/2
- current balance = 128
- 4account = ‘Ali’
- _spam = ‘Ali’
- 42 = ‘amount’
- SPAM = ‘amount’
- Total_$um = 2345
- Account4 = ‘Ali’
- ‘hello’ = ‘world’
Variable names are case-sensitive, meaning that spam, SPAM, Spam, and sPaM are four different variables. It is a Python convention to start your variables with a lowercase letter.
Activity 10:
Write a Python program that uses assignment operators and comments.
Solution:
Type the code given below either in IDLE or as a script. Execute it.
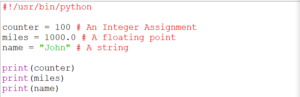
Activity 11:
Combine numbers and text.
Solution:
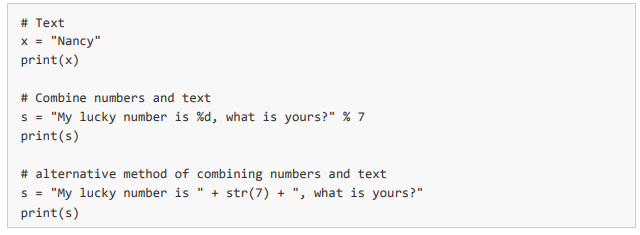
Type the following code. Run it.
Activity 12:
Take input from the keyboard and use it in your program.
Solution:
In Python and many other programming languages you can get user input. In Python the input() function will ask keyboard input from the user. The input function prompts text if a parameter is given. The function reads input from the keyboard, converts it to a string and removes the newline (Enter).Type and experiment with the script below.
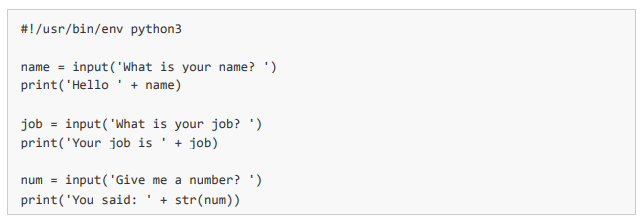
Related links
Microsoft word Installation of Python List and Tuple in python
Power Point Variable and statement String in python
Microsoft Excel If else statement in python functions in python
Microsoft Access loops in python Ubunto operating system
At Cui tutorial, courses, past papers and final year projects
#tutorial #cui #pastpaper #courses