Loop In Python
For and while loops in python
This Lecture teaches you the following topics:
- while loop
- for loop
- Use of break statement
- Nested loops
Introduction:
Loops are one of the basic structures of a programming language. Loops are performed to repeat a step or steps for a certain number of times. Python offers two looping statements called while and for. Both have their own uses and advantages.
This lab session covers both of the loop structures.
while loop
The commonly used syntax of while loop is as below.
while condition:
statement1
statement2
statement3
…
The statements written after the while statements (indented block) are repeated as long as the condition is true. The control will transfer to the statement written outside the indented block when the condition becomes false. We can also break the continuation of the loop by writing break statement within indented block as below.
while condition1:
statement1
statement2
if condition2:
break
statement3
…
In the above case, the while loop will be stopped if the result of condition2 is true.
While loop can also have an optional else part and is executed if break statement was not executed. The syntax of while loop having else block is as below.
while condition:
statement1
statement2
statement3
…
else:
statement1
statement2
statement3
…
for loop
for loop can be used in a number of ways. One of its syntax is as below.
for var in range(value1,value2,value3):
statement1
statement2
statement3
…
value1 refers to the initial value of the range. It will be considered 0 if not mentioned.
Value2 refers to the final value of the range. It will be required to perform the loop.
Value3 refers to the step value. It will be added (incremented) every time the loop is repeated. It will considered 1 if skipped.
for loop has an optional else part as well. It works exactly as in while loop. It is executed only if break statement is not executed. The syntax becomes as below.
for var in range(value1,value2,value3):
statement1
statement2
statement3
…
else:
statement1
statement2
statement3
…
There is also use of for loop to be used with lists. It will be covered in the next lab session.
Activity 1:
Calculate the sum of all the values between 0-10 using for loop.
Solution:
- Create a new Python file from Python Shell and type the following
- Run the code by pressing F5.
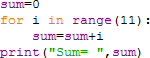
You will get the following output.
Activity 2:
Repeat the above code using while loop.
Solution:
- Create a new Python file from Python Shell and type the following
- Run the code by pressing
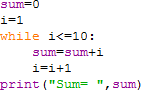
You will get the following output.
Activity 3:
Accept 5 integer values from user and display their sum.
Solution:
- Create a new Python file from Python Shell and type the following
- Run the code by pressing F5.
You will get the following output.
Activity 4:
Write a Python code to keep accepting integer values from user until 0 is entered. Display sum of the given values.
Solution:
- Create a new Python file from Python Shell and type the following
- Run the code by pressing F5.
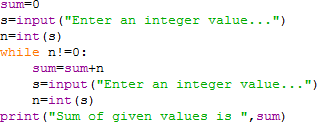
You will get the following output.
Activity 5:
Write a Python code to accept an integer value from user and check that whether the given value is prime number or not.
Solution:
- Create a new Python file from Python Shell and type the following
- Run the code by pressing F5
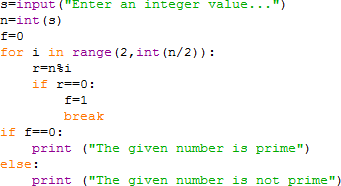
.
You will get the following output.
If we run the program again and enter 17 as input value then the output will be as below.
Home Activities:
Activity 1:
Replace the for loop with while loop in the activity 5 discussed above.
Activity 2:
Write a Python code to display all the prime numbers between 100 to 200.
Activity 3:
Write a program that takes a number from user and calculate the factorial of that number.
Activity 4:
Fibonacci series is that when you add the previous two numbers the next number is formed. You have to start from 0 and 1.
E.g. 0+1=1 → 1+1=2 → 1+2=3 → 2+3=5 → 3+5=8 → 5+8=13
So the series becomes
0 1 1 2 3 5 8 13 21 34 55 ……………………………………
Steps: You have to take an input number that shows how many terms to be displayed. Then use loops for displaying the Fibonacci series up to that term e.g. input no is =6 the output should be
0 1 1 2 3 5
Related links
Microsoft word Installation of Python List and Tuple in python
Power Point Variable and statement String in python
Microsoft Excel If else statement in python functions in python
Microsoft Access loops in python Ubunto operating system
At Cui tutorial, courses, past papers and final year projects
#tutorial #cui #pastpaper #courses