If else Statements in Python
If else Statements in Python Programming
This lecture teaches you the following topics:
- Use of indentation
- Use of simple if statement
- Use of if-else statement
- Use of nested-if statement
Introduction:
- ‘if’ statement is used to perform logical operation.
- In order to perform decision making, we need to check certain condition(s) before processing.
- Python supports if statement for doing so.
- There are various formats of if statement including if-else and if-else if.
If Statement
The basic and shortest form of if statement is as below:
if condition:
statement1
statement2
…
…
- If the condition is true then the specified block will be executed.
- It is important to note that the block is specified by the use of indentation.
- Python does not use a pair of curly bracket { } to specify the block.
Logical Conditions:
- Equals: a == b
- Not Equals: a != b
- Less than: a < b
- Less than or equal to: a <= b
- Greater than: a > b
- Greater than or equal to: a >= b
If-Else Statement
We can also write the else block associated with the if statement as below.
if condition:
statement1
statement2
…
else:
statement3
statement4
…
If-elseif-else statement
If we are required to test a number of conditions and want to execute one of the many blocks of statements, then we can use if-elif-else statement as below.
if condition1
statement1
statement2
…
else if condition2:
Statement3
Statement4
…
…
else if condition3:
Statement5
Statement6
…
…
else:
Statement7
Statement8
Lab Activities:
Activity 1:
Let us take an integer from user as input and check whether the given value is even or not.
Solution:
- Create a new Python file from Python Shell and type the following
- Run the code by pressing
You will get the following output.
Activity 2:
Let us modify the code to take an integer from user as input and check whether the given value is even or odd. If the given value is not even then it means that it will be odd. So here we need to use if-else statement an demonstrated below.
Solution:
- Create a new Python file from Python Shell and type the following
- Run the code by pressing

You will get the following output.
Activity 3:
Let us accept two integer values from user and compare which one is the larger value.
If both are equal values then we should display that both are equal values.
In this activity you will use if-elseif-else structure of if statement.
This is used when we need to check more than one conditions to perform certain action.
Solution:
- Create a new Python file from Python Shell and type the following
- Run the code by pressing F5.
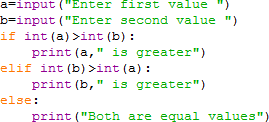
You will get the following output.
Activity 4:
Let us modify the above code in order to apply nested if structure. Sometimes we need to use an if statement within the block of another if to find the solution of the problem. The following code example illustrates that how nested if can be used in Python.
Solution:
- Create a new Python file from Python Shell and type the following
- Run the code by pressing
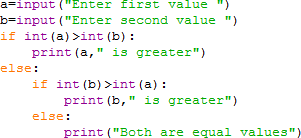
You will get the following output.
Home Activities:
Activity 1:
Write a Python code to accept marks of a student from 1-100 and display the grade according to the following formula.
Grade F if marks are less than 50
Grade E if marks are between 50 to 60
Grade D if marks are between 61 to 70
Grade C if marks are between 71 to 80
Grade B if marks are between 81 to 90
Grade A if marks are between 91 to 100
Activity 2:
Write a Python code to accept temperature value from user (in centigrade) and display an appropriate message as below.
FREEZING if temperature in less than 0
COLD if temperature is between 0 to 15
WARM if temperature is between 16 to 30
HOT if temperature is between 31 to 40
VERY HOT if temperature is greater than 40
Related links
Microsoft word Installation of Python List and Tuple in python
Power Point Variable and statement String in python
Microsoft Excel If else statement in python functions in python
Microsoft Access loops in python Ubunto operating system
At Cui tutorial, courses, past papers and final year projects
#tutorial #cui #pastpaper #courses