Functions in Python
Functions in python
- How to define own functions
- How to use user-defined functions
- Passing different types of arguments
Introduction:
- It is usually a better approach to divide a large code in small functions.
- A function is a small piece of code used to perform a specific purpose.
- Functions are defined first then called whenever needed.
- A program may have as many functions as required.
- Similarly a function may be called as many times as required.
How to Define Functions
Functions are defined as below.
def function_name(list_of_parameters):
statement1
statement2
statement3
…
return value
The list of parameters is optional if a function is not accepting any value but is usually required while defining a function.
Similarly, return statement is optional but is required if a function returns a value.
How to Call A Function
Once a function is defined then it can be called by using its name and providing values to the parameters. Calling a function in Python is same as in other programming languages.
Activity 1:
Define a function to accept an integer value and return its factorial.
Solution:
- Create a new Python file from Python Shell and type the following
- Run the code by pressing
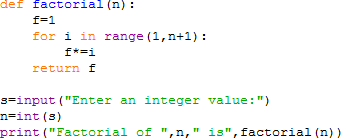
You will get the following output.
Activity 2:
Write a function to accept 2 integer values from user and return their sum.
Solution:
- Create a new Python file from Python Shell and type the following code.
- Run the code by pressing F5.
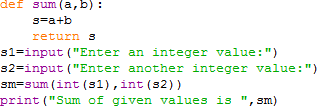
You will get the following output.
Activity 3:
Define a function to accept an integer value from user and check that whether the given value is prime number or not. If the given value is a prime number then it will return true otherwise false.
Solution:
- Create a new Python file from Python Shell and type the following code.
- Run the code by pressing F5.
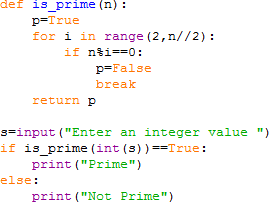
You will get the following output.
Activity 4:
Define a function to accept a list of integer values and return the sum of it.
Solution:
- Create a new Python file from Python Shell and type the following code.
- Run the code by pressing F5.
You will get the following output.
Activity 5:
Write a Python code to accept a list of integers and sort it in descending order.
Solution:
- Create a new Python file from Python Shell and type the following
- Run the code by pressing F5.
You will get the following output.
Home Activities:
Activity 1:
Write a function to accept an integer ‘n’ and display ‘n’ elements of Fibonacci series.
Activity 2:
Write a function to accept two lists of integer values and return the largest value.
Activity 3:
Write a function to accept two integer values and swap them with each other.
Related links
Microsoft word Installation of Python List and Tuple in python
Power Point Variable and statement String in python
Microsoft Excel If else statement in python functions in python
Microsoft Access loops in python Ubunto operating system
At Cui tutorial, courses, past papers and final year projects
#tutorial #cui #pastpaper #courses