If Else statement /conditional structure
—In everyday life, we are often making decision.
—“if the age of the person is above 60, then discount the ticket price to 40%”
—The above statement have element of decision making. E.g only if the age is above 60 reduce the ticket by 40%. If the criteria is not matched the ticket shall not be reduced by 40%.
—This decision making process is everywhere in our daily life.
—Everyday programming requires three control structures:
Control Structures are
- Sequence
- Selection
- Iteration
Sequence
Executing instructions in sequence
Working “down the page”
C / C++ follow this sequence of control until some other is specified via return, if, while, do / while, etc.
Sequential Execution
–Statements executed one after the other in order in which they are written
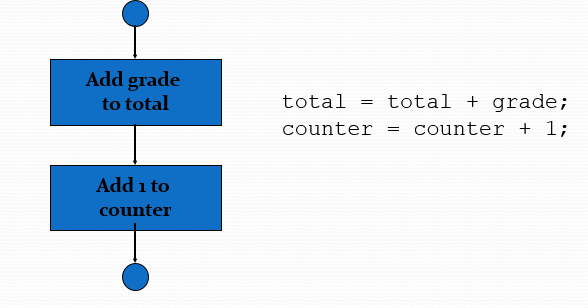
Selection
—Conditional logic
—Choosing between alternative paths based on some predefined test
—Commonly implemented as “if” statements
—May also be implemented in other statements, such as “switch”, “? : “, or embedded within other types of statements such as “for”
—The statement used for decisions in C++ language is known as the ‘if statement ‘.
—If statement has a simple structure and it helps to choose among alternative courses of actions.
Structure of if statement
if (condition)
statement (or group of statements)
-The above statement means , if condition is true, then execute the statement or a group of statements.
-Here the condition is a statement which explains the condition on which a decision will be made.
-Example: your grade and GPA
if ( the grade is greater than or equal to 60)
pass the student
- if selection structure
–Performs an indicated action when the condition is true.
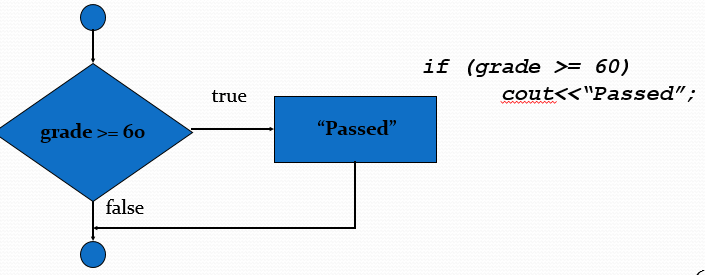
—We write the condition in parentheses, followed by a statement of group of statements to be executed.
—We use braces { } to make a group (block) of a number of statements.
—We put ‘{‘ before the first statement and ‘}’ after the last statement.
if (condition)
{
statement;
statement;
.
.
statement;
}
// if statement is only executed when the value is true
Example: Comparing the ages of two students. If the age of first student is greater than second, then display “ student 1 is older”
main()
{
int age1, age2;
age1 = 12;
age2 = 10;
if (age1 > age2)
cout<<“Student1 is older:;
}
Relational Operators
Relational Operator Meaning
== is equal to
< is less than
> is greater than
<= is less than or equal to
>= is greater than or equal to
!= is not equal to
if/else Structure
—if structure executes its block of statements only when the condition is true, otherwise the statement is skipped.
—The if/else structure allows the programmer to specify that a different block of statement(s) is to be executed when the condition is false.
- if/else selection structure
–Performs an action when the condition is true and another when the condition is false.

if (condition)
{
statement(s)
}
else
{
statement (s)
}
Example
if (age1 > age2)
{
cout<<“ student1 is older”;
}
else
{
cout<< “ student2 is older”;
}
—What if student1 and student2 are of the SAME age???
Use >= operator
Q. Write a program in which users enter a number. If the user enter positive number, add one to the number else add two to the number.
Program
main()
{
int num, neg ,pos;
cout<<“Please enter the no.”;
cin>> num;
if (num > 0)
pos= pos +1;
else
neg= neg +2;
}
Conditional Operator
- Closely related to if/else structure
- ?: (Conditional Operator)
- Only ternary operator in C
–Takes three operands
- Operands with conditional operator form conditional expression
–Operand 1– condition
–Operand 2 – action if condition true
–Operand 3 – action if condition false
Conditional Operator
grade >= 60 ? “Passed”: “Failed”
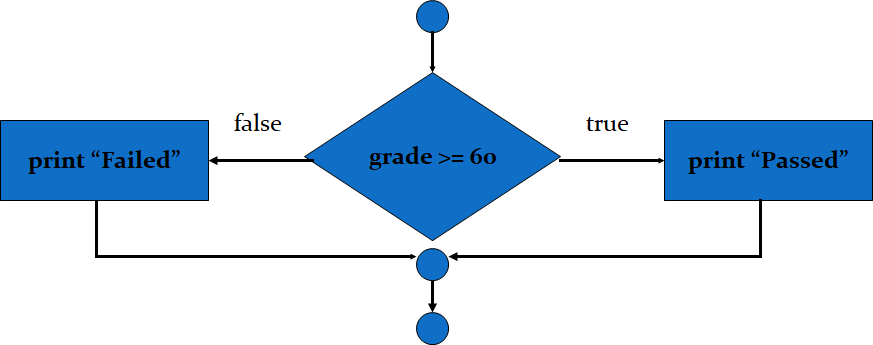
Nested if Statements
—Used for multiple conditions and take some actions accordingly to each condition. Example, Grade, payroll etc.
—In this case we use NESTED IF statement.
if (condition 1 )
Statement1
else if (condition 2 )
Statement2
…
else if (condition N )
StatementN
else
Statement N+1
EXACTLY 1 of these statements will be executed.
Example of grades (if only)
if (grade==‘A’)
cout<<“Excellent”;
if (grade==‘B’)
cout<<“Very Good”;
if (grade==‘C’)
cout<<“Good”;
if (grade==‘D’)
cout<<“poor”;
if (grade==‘F’)
cout<<“Fail”;
—‘if Statement” is computationally one of most expensive statements in program due to the fact that processor has to go through many cycles to execute them for a single decision.
—To avoid this , and alternate of multiple if statements can be use of if/else statements.
Nested if/else
//nested if/else
//printing remarks for each grade
if (grade == ‘A’)
cout<<“Excellent”;
else
if (grade == ‘B’)
cout<<“Very Good”;
else
if (grade == ‘C’)
cout<<“Good”;
else
if (grade == ‘D’)
cout<<“Poor”;
else
cout<<“Failed”;
//nested if/else
//printing grades of students
if (grade >= 90)
cout<<“A”;
else
if (grade >= 80)
cout<<“B”;
else
if (grade >= 70)
cout<<“C”;
else
if (grade >= 60)
cout<<“D”;
else
cout<<“Failed”;
//nested if/else
//printing grades of students
if (grade >= 90)
cout<<“A”;
else if (grade >= 80)
cout<<“B”;
else if (grade >= 70)
cout<<“C”;
else if (grade >= 60)
cout<<“D”;
else
cout<<“Failed”;
Feature of Nested if/else
—In the ‘nested if statements’ the nested else is not executed if the first if condition is true and the control goes out of the if block.