Relationship Between Pointers and Arrays
Relationship Between Pointers and Arrays
- You can use the expression ptr++ for a pointer variable
- But You cannot use the ++ operation if the pointer is the name of an array
- It is a constant pointer and can’t be changed.
Comparing pointer
- Write a program to input an array of size 10 and then display the array using pointer to array.
int num[10];
int *start, *end;
start = num;
end = &num[9];
……
……
int main() {
int num[10];
int *start, *end;
start = num;
end = &num[9];
while(start<=end) {
cout << “Enter a number: “;
cin >> *start;
start++;
}
start = num; /* reset the starting pointer */
while(start<=end) {
cout << *start << ‘ ‘;
start++;
}
return 0; }
Arrays of Pointers
int *pi[10];
int var;
pi[2] = &var; /* assign address of an integer var to third element of pointer array */
To find value of var:
*pi[2]
int *b[5];
int c[10]={0};
int f[5]={0};
b[2]=c;
b[1]=&f[0];
for(int I =0; I<5;I++)
b[1][I] = I;
for(int I =0; I<5;I++)
cout<<f[I];
for(int I =0; I<10;I++)
b[2][I] = I;
for(int I =0; I<10;I++)
cout<<c[I];
int *b[5];
int c[10]={0};
int f[5]={0};
b[2]=c;
b[1]=&f[0];
for(int I =0; I<5;I++)
b[1][I] = I;
for(int I =0; I<5;I++)
cout<<f[I]; // values in f are changed through b[1][i]// 0,1,2,3,4
for(int I =0; I<10;I++)
b[2][I] = I; // changes values in array to which pointer at b[2] is pointing i.e c
for(int I =0; I<10;I++)
cout<<c[I];
Pointer passing to function
bool stringcopy (char *, char *, char *);
stringcopy(string1, string2,str3)//function call
bool stringcopy (char * str1, char *str2, char * str3) //definition
Void int_operation (int *, int *);
Why use pointers
- Passing value to function by reference
- Dynamic memory allocation
- Size of array must be known at the compile time.
- Size cannot be variable.
- If size if unknown, we need to declare a pointer and allocate memory using new[]
- Dynamic memory allocation
int size=10;
int *array1 = new int [size];
array1[3]=23;
array1[0]=100;
cout<<array1[0]<<” “<<*(array1+3) ;
Exercise
- Write a function that calculates the size of a character array and returns the string length.
- Parameter(s) are passed by pointers.
- You cannot use the array notation[], except for the initial declaration.
String length function
int strlen(char *strptr)
{
int count=0;
while (*(strptr+count)!=’\0′)
{
count++;
}
return count;
}
Dynamic Memory Deallocation
- We need to deallocate memory that is dynamically allocated
- Mainly good to do so
- Memory stays “reserved” even if you’re not actually using it
- Also at times necessary or you’ll run out of memory
int *array1 = new int [size];
delete [] array1; // delete the array pointed to by pointer array1
int *ptr = new int
delete ptr; // delete the variable pointed to by pointer ptr
Creating a dynamic 2D array
int columnsize=4
int *arrayptr[3];
arrayptr[0]=new int [columnsize];
arrayptr[1]=new int [columnsize];
arrayptr[2]=new int [columnsize];
- Here we are creating the 2D array columns dynamically but the row is fixed to 3
- We need to create the array of pointers dynamically as well
int row=2,col=3;
int **ptr= new int *[row];// dynamically creating 2d array
// create a pointer to array of pointers
// array of pointers is dynamically created
// for each index of pointer array create a dynamic array
for (int i=0;i< row;i++)
ptr[i]=new int [col];
Homework
- Create 2 2D matrices (ask the user for dimensions) and perform the addition on both matrices.
Array of strings
// array of strings
#include <iostream>
using namespace std;
int main()
{
const int DAYS = 7; //number of strings in array
const int MAX = 10; //maximum size of each string
//array of strings
char star[DAYS][MAX] = { “Sunday”, “Monday”, “Tuesday”,
“Wednesday”, “Thursday”,
“Friday”, “Saturday” };
for(int j=0; j<DAYS; j++) //display every string
cout << star[j] << endl;
return 0;
}
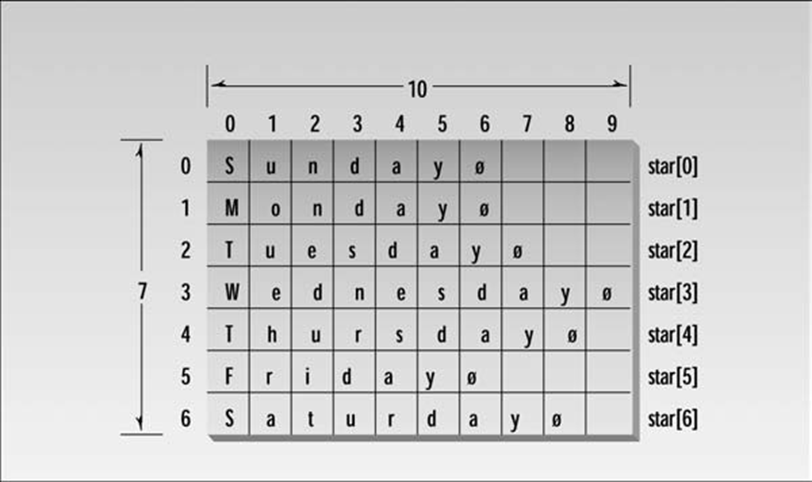
- Space wasted because of 2D array fixed column length
- Use array of pointers instead
const int DAYS = 7; //number of pointers in array
int main()
{ //array of pointers to char
char* arrptrs[DAYS] = { “Sunday”, “Monday”, “Tuesday”,
“Wednesday”, “Thursday”,
“Friday”, “Saturday” };
for(int j=0; j<DAYS; j++) //display every string
cout << arrptrs[j] << endl;
return 0;
}