Pointers and Arrays
Pointers and Arrays
Pointer Expressions and Pointer Arithmetic
- When you place an asterisk after a data type, you mean the data type points to something. When you place an asterisk in front of a variable name, you are referring to the contents of that address.
- The computer must know what type of variable a pointer points to so the compiler can increment the pointer to the next location of that type of variable
- Pointer arithmetic
- Increment/decrement pointer (++ or —)
- Add/subtract an integer to/from a pointer( + or += , – or -=)
- Pointers may be subtracted from each other
- Pointer arithmetic meaningless unless performed on pointer to array
-
- 5 element int array on a machine using 4 byte ints
- vPtr points to first element v[ 0 ], which is at location 3000
vPtr = 3000
- vPtr += 2; sets vPtr to 3008
vPtr points to v[ 2 ]
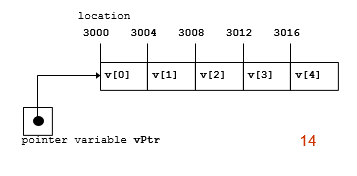
- Subtracting pointers
- Returns number of elements between two addresses
vPtr2 = v[ 2 ];
vPtr = v[ 0 ];
vPtr2 – vPtr == 2
- Pointer assignment
- Pointer can be assigned to another pointer if both of same type
- Arrays and pointers closely related
- Array name like constant pointer
- Pointers can do array subscripting operations
- Accessing array elements with pointers
- Element b[ n ] can be accessed by *( bPtr + n )
- Called pointer/offset notation
- Addresses
- &b[ 3 ] same as bPtr + 3
- Array name can be treated as pointer
- b[ 3 ] same as *( b + 3 )
- Pointers can be subscripted (pointer/subscript notation)
- bPtr[ 3 ] same as b[ 3 ]
Relationship Between Pointers and Arrays
#include <iostream.h>
int main ()
{
int numbers[5]; int * p;
p = numbers; *p = 10;
p++; *p = 20;
p = &numbers[2]; *p = 30;
p = numbers + 3; *p = 40;
p = numbers; *(p+4) = 50;
for (int n=0; n<5; n++)
cout << numbers[n] << “, “;
return 0; }
int main()
{
int b[] = { 10, 20, 30, 40 };
int *bPtr = b; // set bPtr to point to array b
// output array b using array subscript notation
cout << “Array b printed with:\n”
<< “Array subscript notation\n”;
for ( int i = 0; i < 4; i++ )
cout << “b[” << i << “] = ” << b[ i ] << ‘\n’;
// output array b using the array name and
// pointer/offset notation
cout << “\nPointer/offset notation where ”
<< “the pointer is the array name\n”;
for ( int offset1 = 0; offset1 < 4; offset1++ )
cout << “*(b + ” << offset1 << “) = ”
<< *( b + offset1 ) << ‘\n’;
// output array b using bPtr and array subscript notation
cout << “\nPointer subscript notation\n”;
for ( int j = 0; j < 4; j++ )
cout << “bPtr[” << j << “] = ” << bPtr[ j ] << ‘\n’;
cout << “\nPointer/offset notation\n”;
// output array b using bPtr and pointer/offset notation
for ( int offset2 = 0; offset2 < 4; offset2++ )
cout << “*(bPtr + ” << offset2 << “) = ”
<< *( bPtr + offset2 ) << ‘\n’;
return 0; // indicates successful termination
} // end main
OUTPUT
- You can use the expression ptr++ for a pointer variable
- But You cannot use the ++ operation if the pointer is the name of an array
- It is a constant pointer and can’t be changed.
2D Arrays
- Two dimensional arrays are accessed in a row-column matrix.
- First index indicates the row and the second indicates the column
- bytes = row x column x number of bytes in type
Multiple-Subscripted Arrays
- To initialize
- Initializes grouped by row in braces
int b[2][2]= {{ 1, 2 },{ 3, 4 } };
-
-
- Row 0 Row 1
-
int b[2][2]= {{ 1 }, { 3, 4 } };
#include <iostream.h>
void main()
{ int array1[ 2 ][ 3 ] = { { 1, 2, 3 }, { 4, 5, 6 } };
int array2[ 2 ][ 3 ] = { 1, 2, 3, 4, 5 };
int array3[ 2 ][ 3 ] = { { 1, 2 }, { 4 } };
//Note the various initialization styles. The elements in array2 are assigned to the first row and then the second.
cout << “Values in array1 by row are:” << endl;
for ( int i = 0; i < 2; i++ ) { // for each row
for ( int j = 0; j < 3; j++ ) // output column values
cout << array1[ i ][ j ] << ‘ ‘;
cout << “Values in array2 by row are:” << endl;
for ( int i = 0; i < 2; i++ ) { // for each row
for ( int j = 0; j < 3; j++ ) // output column values
cout << array2[ i ][ j ] << ‘ ‘;
cout << “Values in array3 by row are:” << endl;
for ( int i = 0; i < 2; i++ ) { // for each row
for ( int j = 0; j < 3; j++ ) // output column values
cout << array3[ i ][ j ] << ‘ ‘;
return 0;
} // end main
OUTPUT
Values in array1 by row are:
1 2 3
4 5 6
Values in array2 by row are:
1 2 3
4 5 0
Values in array3 by row are:
1 2 0
4 0 0
Accessing 2-D arrays with pointers
int main()
{
int numbers[5][5]={0};int *p;
p = numbers[0]; // pointing to array numbers [0]
*p = 10;
*(p+1)=20;
*(p+2)=30;
*(p+3)=40;
*(p+4)=50;
for (int i=0;i<5;i++)
cout<<numbers[0][i]<<“ “;
return 0;
}
Output
10 20 30 40 50
int A2[2][3]={{1,2,3},{4,3,5}};
int *A2ptr=A2[0];
A2ptr now points to the array at index 0 of A2 which is {1,2,3}
A2ptr[0]=?
A2ptr[1]=?
A2ptr[2]=?