Data Types in C++
- Unsigned Integers
- Signed Integers
- Floating point numbers
- Characters
- Logical values
Standard Data Types in C++
Following are examples of predefined data types used in C++
int an integer number (e.g. 10, -5).
float a real number (e.g. 3.1415, 2.1).
char a character (e.g. ‘a’, ‘C’).
bool Logical value (true or false)
Integer
The integers type comes in these sizes:
–short int – 2 bytes: (-32,768 to 32,767)
–“plain” int – 4 bytes: (-2,147,483,648 to 2,147,483,647)
The integer type comes in two forms
–signed
–unsigned
“plain” int are always signed
Floating-point Types
They express numbers with decimals and/or exponents.
They come in 3 sizes
Examples:
–3.14159 // 3.14159
–6.02e23 // 6.02 x 10-23
–1.6e-19 // 1.6 x 10-19
–3.0 // 3.0
Boolean
bool can have value true or false.
Represent logical result
Example
bool IsPrime= false;
bool a = true;
bool b = true;
bool x = a + b; //a+b = 2, so x becomes true
bool y = a | b; //a | b is 1, so y becomes true;
Character
- char ch =‘a’;
- 0-127 are standard ASCII characters
- Every character constant has an integer value
- For Example, A = 65
- A=97
Data Representation
What is coding system?
- To represent numeric, alphabetic, and special characters in a computer’s internal storage and on magnetic media, we must use some sort of coding system
- A unique code for each decimal value (0 through 9), each uppercase and lowercase letter, and for a variety of special characters
ASCII coding system—American Standard Code for Information Interchange:
- 8 bit code to represent different characters
How to represent character?
How can an alphabet be stored in computer?
Decimal equivalent of characters
–A = 65
–B= 66
–C= 67
.
.
.
–a=97
In ASCII coding system
ASCII table
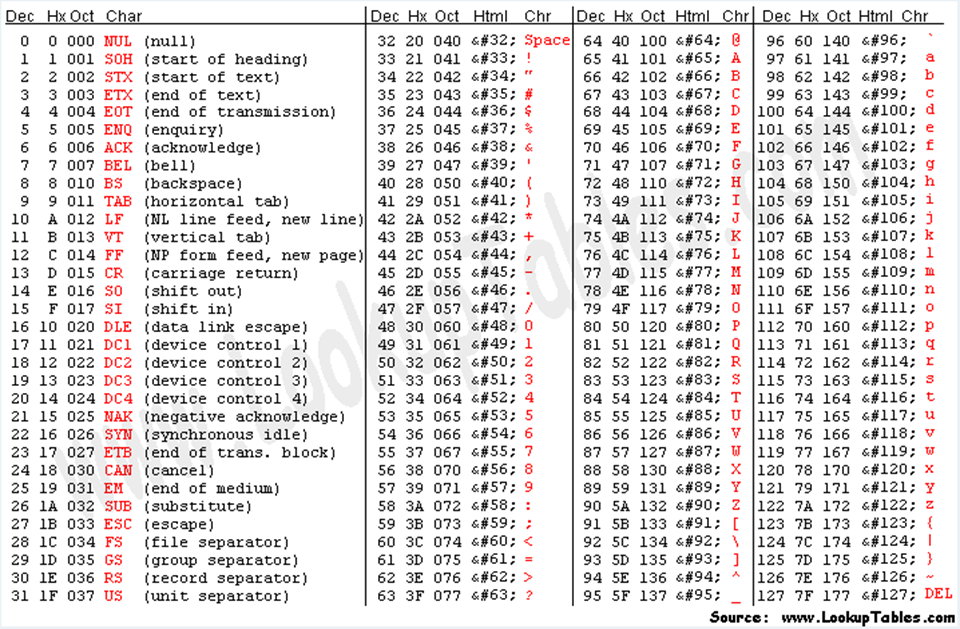
size of operator
Sizeof operator
- Returns size of data items in bytes
- For example, sizeof (int) = 4 bytes
int main()
{ char c; short s; int i; long l; float f; double d; long double ld; int array[ 20 ];
cout << “sizeof c = “ << sizeof (c)
<< “\tsizeof(char) = “ << sizeof( char )
<< “\nsizeof s = ” << sizeof (s)
<< “\tsizeof(short) = “ << sizeof( short )
<< “\nsizeof i = “ << sizeof (I )
<< “\tsizeof(int) = “ << sizeof( int )
<< “\nsizeof l = “ << sizeof (l )
<< “\tsizeof(long) = “ << sizeof( long )
<< “\nsizeof f = “ << sizeof (f )
<< “\tsizeof(float) = “ << sizeof( float )
<< “\nsizeof d = “ << sizeof (d )
<< “\tsizeof(double) = ” << sizeof( double )
<< “\nsizeof ld = ” << sizeof ( ld)
<< “\tsizeof(long double) = “ << sizeof( long double )
<< “\nsizeof array = “ << sizeof (array)
<< endl;
return 0; // indicates successful termination } // end main
Operator sizeof can be used on variable name.
Operator sizeof can be used on type name.
sizeof c = 1 sizeof(char) = 1
sizeof s = 2 sizeof(short) = 2
sizeof i = 4 sizeof(int) = 4
sizeof l = 4 sizeof(long) = 4
sizeof f = 4 sizeof(float) = 4
sizeof d = 8 sizeof(double) = 8
sizeof ld = 8 sizeof(long double) = 8
sizeof array = 80
What is the largest integer value that can be expressed in 32 bits?
- How to calculate range?
- [0…(2n – 1)]
- For 2 bits?
- 8 bits?
- For 32-bit computers
from 0 to 4,294,967,295
- For 32-bit computers
- Integer range is from -2,147,483,648 to 2,147,483,647
- Integer overflow and underflow
What will be the output?
int a=2147483647;
cout<<++a;
int a=-2147483648;
cout<<a–;
cout<<” “<<a;
What will be the output?
int a=2147483647;
cout<<++a; //-2147483648 overflow
int a=-2147483648;
cout<<a–; //-2147483648
cout<<” “<<a; //2147483647 underflow