Methods Overloading and Recursion in Java
Activity Outcomes:
This lab teaches you the following topics:
Methods overloading
Recursion
Introduction:
Methods overloading means to define more than one methods having same name but different signatures in the same class. It enables us to use the same method’s name multiple time in the same class. The functionality of each method may be different. It is important to note that the return type of the method has nothing to do with
overloading. The return type may be kept same or different but the number or type of parameters must be different to implement methods overloading. Sometimes it may be required that a method should call itself to solve a particular problem. If this happens then this is called recursion.
Methods Overloading
The important points in methods overloading are as below.
1. All the methods involved in overloading must be defined in the same class.
2. Names of all the methods must be same.
3. Number of parameters should be different.
4. If number of parameters are same then data type of at least on of them should be different from other methods.
5. Names of parameters do no matter in methods overloading
6. Return type of methods may be same or different as return type is also not considered in methods overloading
Recursion
In recursion, a method calls itself. If a method starts calling itself then a kind of loop starts to happen. You must write some statements to break this loop so recursion stops.
Lab Activities:
Activity 1:
Write a Java program to have two methods of same name called sum. One of them should accept two integer values and return their sum. The other sum method should accept three integer values and return their sum.
Solution:
Create a new Java file and type the following code.
Run the code by pressing F5.
You will get the following output.
Here is the modified to code call the method having three parameters.
The output will be as below.
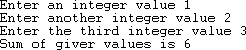
Activity 2:
Write two methods having name sum. One of them will add two integer values and the other will add two double values.
Solution:
Create a new Java file and type the following code.
Run the code by pressing F5.
You will get the following output.
Activity 3:
Write two methods having name max. One of them will accept an array of integers and return maximum value. The other will accept an array of strings and return the string having maximum number of characters in it.
Solution:
Create a new Java file and type the following code.
Run the code by pressing F5.
You will get the following output.
Activity 4:
Write a method to find factorial of an integer using recursion.
Solution:
Create a new Java file and type the following code.
Run the code by pressing F5.
You will get the following output.
Activity 5:
Write a Java program to display Fibonacci series by using a recursive method.
Solution:
Create a new Java file and type the following code.
Run the code by pressing F5.
You will get the following output.
Home Activities:
Activity 1:
Write the following overloaded methods and use them in a Java program.
int multiply(int a, int b)
double multiply(double x, double y)
float multiply(float a, float b)
Activity 2:
Write two overloaded methods having name sort. One of them will accept an array of strings and sort them in descending order. The other will accept an array of integer values and will sort them in descending order.
Activity 3:
Write a recursive method to build tower of Hanoi.