Loops in Java
Activity Outcomes:
This lab teaches you the following topics:
for loop
nested for loop
Use of break statement in for loop
Introduction:
Loops are one of the basic structures of a programming language. Loops are performed to repeat a step or steps for a certain number of times. Java offers a number of loop statements. One of them is for loop. This lab session covers the for loop.
for loop
for loop is probably the most commonly used loop in Java. It is preferred to use in situations when the number of iterations are known in advance, i.e. we already know that how many times the loop will be repeated.
Syntax
The syntax of for loop is as below.
for(exp1;exp2;exp3)
statement;
or
for(exp1;exp2;exp3)
{
statement1;
statement2;
statement3;
…
…
}
Where, exp1 is called initialization. Here we initialize the variable to be used to control the loop iterations.
exp2 is a condition. Loop will continue to iterate as long as this condition is true.
exp3 is an increment or decrement statement. It will increment or decrement the loop variable initialized in exp1.
Lab Activities:
Activity 1:
Calculate the sum of all the values between 0-10 using for loop.
Solution:
Create a new Java and type the following code.
Run the code by pressing F5.
You will get the following output.
Activity 2:
Accept an integer values from user and display its numeric table.
Solution:
Create a new Java file and type the following code.
Run the code by pressing F5.
You will get the following output.
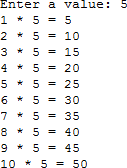
Activity 3:
Accept an integer values from user and display its factorial.
Solution:
Create a new Java file type the following code.
Run the code by pressing F5.
You will get the following output.

Activity 4:
Write a Java code to accept an integer value from user and check that whether it is prime or not. In this activity, you will also learn the use of break statement in for loop.
break statement is used in for loop to terminate the for loop immediately and the next iterations will not be performed as they may not be required further.
Solution:
Create a new Java file and type the following code.
Run the code by pressing F5.
You will get the following output.
Activity 5:
In this activity, you will learn the use of nested for statements. If we use a for statement within the body of another for statement then it is called nested for statements. You are required to write a Java program to display all the prime numbers between 100 to 200.
Solution:
Create a new Java file and type the following code.
Run the code by pressing F5.
You will get the following output.

Home Activities:
Activity 1:
Write a Java program to accept 10 integer values from user and display sum of positive values.
Activity 2:
Write a Java program to generate the following output.
Activity 3:
Write a Java program that prompts the user to enter two positive integers and find their greatest common divisor as below.
Activity 4:
Fibonacci series is that when you add the previous two numbers the next number is formed. You have to start from 0 and 1.
E.g. 0+1=1 → 1+1=2 → 1+2=3 → 2+3=5 → 3+5=8 → 5+8=13
So the series becomes
0 1 1 2 3 5 8 13 21 34 55 ……………………………………
Steps: You have to take an input number that shows how many terms to be displayed.
Then use loops for displaying the Fibonacci series up to that term e.g. input no is =6 the output should be
0 1 1 2 3 5