Conditional statements(if, if-else,Nested if,Switch) in Java
Activity Outcomes:
This lab teaches you the following topics:
Use of indentation
Use of simple if statement
Use of if-else statement
Use of nested-if statement
Use of switch statement
Introduction:
if statement is used to perform logical operation. In order to perform decision making, we need to check certain condition(s) before processing. Java supports if statement for doing so. There are various formats of if statement including if-else and if-else-if.
Apart from if statement, Java also supports switch statement. switch statement is used to select one of the various options.
If Statement:
The basic and shortest form of if statement is as below:
if (condition)
statement;
If the condition is true in above case then the statement written after the if statement will be executed otherwise the given statement will be simple ignored. If there are more than one statements to be executed when the condition is true then we need to write those statements in a pair of curly brackets as below.
if (condition)
{
statement1;
statement2;
…
…
}
If the condition is true then the specified block of statements written within a pair of curly brackets will be executed. It is important to note that the block is specified by the use of a pair of curly brackets.
We can also write the else block associated with the if statement as below.
if (condition)
statement1;
else
statement2;
The else part of if statement is always optional but if is written then will be executed only if the condition written after the if statement is false. In above case if the condition is true then statement1 will be executed and statement2 will be ignored. On the other hand, if the condition written after the if statement is false then statement2 will be executed and statement1 will be ignored.
We can also write more than one statements in the if block or in the else block as shown below.
if (condition)
{
statement1;
statement2;
…
…
}
else
{
statement3;
statement4;
…
…
}
If we are required to test a number of conditions and want to execute one of the many blocks of statements, then we can use multi-way if-else statements as below.
if (condition1)
{
statement1;
statement2;
…
…
}
else if (condition2)
{
statement3;
statement4;
…
…
}
else if (condition3)
{
statement5;
statement6;
…
…
}
else
{
statement7;
statement8;
}
In the above case only one of the written blocks will be executed depending on the condition to be true. If none of the conditions is true then the block written after the last else will be executed. If we have not written the last block then nothing will be executed if all the conditions are false.
Java supports another statement as alternative of the above case, called switch statement as discussed below:
switch statement
The syntax of switch statement is as below.
switch (variable/expression)
{
case value1:
statement1;
statement2;
…
…
break;
case value2:
statement3;
statement4;
…
…
break;
case value3:
statement5;
statement6;
…
…
break;
default:
statement7;
statement8;
…
…
break;
}
In switch statement, only one of the case block can be executed depending on the value of the variable or expression written after the switch statement. Every case block will have a unique value and will be executed if the value hold by the switch variable (or expression) matches that case value.
The break statement is important to write in each case block. It takes control out of the switch statement. If break statement is not written in any case block then the following case block will be executed until break statement is found or the last statement written in the switch statement is executed.
The default block is optional and will be only executed if none of the case value is equal to the value contained of the switch variable (or expression).
Lab Activities:
Activity 1:
Let us take an integer from user as input and check whether the given value is even or not.
Solution:
A. Create a new Java file using an appropriate IDE and type the following code.
B. Run the code.
You will get the following output.

Activity 2:
Let us modify the code to take an integer from user as input and check whether the given value is even or odd. If the given value is not even then it means that it will be odd. So here we need to use if-else statement an demonstrated below.
Solution:
A. Create a new Java file using an appropriate IDE and type the following code.
B. Run the code by pressing F5.
You will get the following output.
Activity 3:
Let us modify the above code in order to apply nested if structure. Sometimes we need to use an if statement within the block of another if to find the solution of the problem. The following code example illustrates that how nested if can be used in Java.
Solution:
A. Create a new Java file and type the following code.
B. Run the code by pressing F5.
You will get the following output.
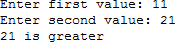
Activity 4:
The code written in activity 3 can also be written as multi-way if-else statement and is in fact a preferred way of writing multiple if-else statements in order to make the code more readable.
Solution:
A. Create a new Java file and type the following code.
B. Run the code by pressing F5.
You will get the following output.
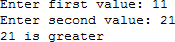
Activity 5:
In this activity, you will be using multi-level if-else statement. You are required to accept two integer values from user and calculate their addition, subtraction, multiplication and division depending on the given choice.
Solution:
A. Create a new Java file and type the following code.
B. Run the code by pressing F5.
You will get the following output.
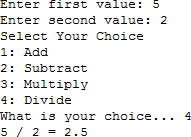
Activity 6:
In this activity, you will rewrite the above code by using switch statement instead of using multi-level if-else statement. You are required to accept two integer values from user and calculate their addition, subtraction, multiplication and division depending on the given choice.
Solution:
A. Create a new Java file and type the following code.
B. Run the code by pressing F5.
You will get the following output.
Home Activities:
Activity 1:
Write a Java code to accept marks of a student from 1-100 and display the grade according to the following formula.
Grade F if marks are less than 50
Grade E if marks are between 50 to 60
Grade D if marks are between 61 to 70
Grade C if marks are between 71 to 80
Grade B if marks are between 81 to 90
Grade A if marks are between 91 to 100
Activity 2:
Write a Java code to accept temperature value from user (in centigrade) and display an appropriate message as below.
FREEZING if temperature in less than 0
COLD if temperature is between 0 to 15
WARM if temperature is between 16 to 30
HOT if temperature is between 31 to 40
VERY HOT if temperature is greater than 40