Functions/Methods in Java
Activity Outcomes:
This lab teaches you the following topics:
How to define own methods
How to use user-defined methods
Passing different types of arguments
Introduction:
It is usually a better approach to divide a large code in small methods. A method is a small piece of code used to perform a specific purpose. Methods are defined first then called whenever needed. A program may have as many methods as required. Similarly a method may be called as many times as required.
How to Define Methods
Functions are defined as below.
returnType methodName(dataType par1, dataType par2, …)
{
statement1;
statement2;
…
return value;
}
A method may or may not return a value. If a method does not return a value then the return type must be void otherwise you need to return the data type of the value to be returned.
A method must have an appropriate name and a pair of parenthesis. In parenthesis, you need to mention that whether a method will accept or not any parameters. If a method is not accepting any parameter then the parenthesis must be left blank otherwise you need to mention the name and type of parameters to be accepted.
A method must return a value if the return type is not void.
How to Call A Function
Once a method is defined then it can be called by using its name and providing values to the parameters. The following activities demonstrate that how methods are defined and called in Java.
Lab Activities:
Activity 1:
Define a method to accept an integer value and return its factorial.
Solution:
Create a new Java file and type the following code.
Run the code by pressing F5.
You will get the following output.
Activity 2:
Write a function to accept 2 integer values from user and return their sum.
Solution:
Create a new Java file and type the following code.
Run the code by pressing F5.
You will get the following output.

Activity 3:
Define a function to accept an integer value from user and check that whether the given value is prime number or not. If the given value is a prime number then it will return true otherwise false.
Solution:
Create a new Java file and type the following code.
Run the code by pressing F5.
You will get the following output.

Activity 4:
Define a function to accept an integer value and display its numeric table.
Solution:
Create a new Java file and type the following code.
Run the code by pressing F5.
You will get the following output.
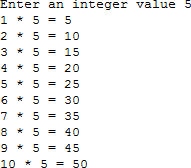
Activity 5:
Write a Java program to find the sum of integers from 1 to 10, from 11 to 20, and from 21 to 30, respectively.
Solution:
Create a new Java file and type the following code.
Run the code by pressing F5.
You will get the following output.
Home Activities:
Activity 1:
Write a method to accept an integer ‘n’ and display ‘n’ elements of Fibonacci series.
Activity 2:
Write a method to accept three integer values and return the largest value.
Activity 3:
Write a method to accept marks of a student (between 0-100) and return the grade according to the following criteria.
0 – 49 F
50 – 60 E
61 – 70 D
71 – 80 C
81 – 90 B
91 – 100 A